DirectX Ops Script Commands
The DirectX Ops tool (see DirectX Ops (dxops.exe)) can accept an expression string, which contains a series of script commands, files and optional arguments. Each expression is built from one or more of the following script commands:
I/O Script Commands |
Description |
load |
Load a model or texture file |
save |
Save model or texture to file |
unload |
Remove textures or models from DXOps |
Mesh Script Commands |
Description |
addvdata |
Add vertex elements to a mesh vertex stream |
clean |
Clean mesh |
clonevdata |
Adds vertex elements to the vertex streams |
delvdata |
Deletes vertex elements to a mesh vertex stream |
flatten |
Removes hierarchy from mesh |
genadjacency |
Generates adjacency data for mesh |
gennormals |
Generates normals for mesh |
gentangentframes |
Generates tangent frames for mesh |
meshes |
Print information about loaded meshes |
optimize |
Optimize meshes |
reset |
Resets DXOps |
stripify |
Convert a mesh into triangle strips |
uvatlas |
Creates UVAtlas for mesh |
validate |
Validate mesh |
Texture Script Commands |
Description |
genmipmaps |
Generates mipmaps for texture(s) |
newtex2d |
Creates a new texture |
newtex3d |
Creates a new volume texture |
newtexcube |
Creates a new cube texture |
reformat |
Changes texture format |
resize |
Resizes the texture |
shade |
Applies a shader to a texture |
slice2d |
Creates a new 2D texture from an existing texture surface |
splice2d |
Inserts a surface into an existing texture |
textures |
Print information about loaded textures |
The script commands can be entered into the tool as command line arguments or from a script file. Each command has zero or more arguments passed in as name-value pairs, with the name and the value separated by a colon. All commands are either I/O or perform some operation on the loaded meshes. Almost every script command has corresponding API(s) in the DirectX SDK that do almost the exact same thing.
addvdata Script Command
Description:
Adds vertex elements to the vertex streams associated with the meshes.
Syntax:
addvdata src:string type:string usage:string usageIdx:int;
Where:
- src:string - Specifies which currently loaded objects to perform this operation on. This string can optionally use .NET Framework Regular Expressions. The default is ".*", meaning perform this operation on all currently loaded objects.
- type:string - The data type. Use the values in D3DDECLTYPE without the D3DDECLTYPE_ prefix (for example: type:FLOAT3). There is no default value. This argument is required and can be unnamed (i.e. passed in as 'FLOAT3' instead of type:'FLOAT3').
- usage:string - The name of the element. Use the values in D3DDECLUSAGE without the D3DDECLUSAGE_ prefix (for example: usage:TANGENT). The default value is TEXCOORD.
- usageIdx:int - Int value used for the usage index of the new vertex declaration entry. The default value is the first valid index for the specified usage.
Example:
addvdata type:FLOAT3 usage:TANGENT usageIdx:0;
Remarks:
The new element is appended to the end of the vertex declaration and to the end of each vertex in the vertex buffer. Note that this command will not initialize any new data added to each vertex. For example, if adding normals a call to gennormals is needed to generate valid normal values for each vertex after they have been added to the declaration.
clean Script Command
Description:
Clean the mesh (or meshes).
Syntax:
clean src:string;
Where:
- src:string - Specifies which currently loaded objects to perform this operation on. This string can optionally use .NET Framework Regular Expressions. The default is ".*", meaning perform this operation on all currently loaded objects.
Example:
clean src:tiger;
Remarks:
Clean calls validation internally; if validation fails, the mesh cannot be cleaned.
Cleans the following issues with the mesh:
- Backfacing - Merge triangles that share the same vertex indices but have face normals pointing in opposite directions (back-facing triangles). Unless the triangles are not split by adding a replicated vertex, mesh adjacency data from the two triangles may conflict.
- Bowties - If a vertex is the apex of two triangle fans (a bowtie) and mesh operations will affect one of the fans, then split the shared vertex into two new vertices. Bowties can cause problems for operations such as mesh simplification that remove vertices, because removing one vertex affects two distinct sets of triangles.
This script command uses D3DXCleanMesh.
clonevdata Script Command
Description:
Adds vertex elements to the vertex streams associated with the meshes.
Syntax:
clonevdata src:string usage:string usageIdx:int newUsage:string newUsageIdx:int;
Where:
- src:string - Specifies which currently loaded objects to perform this operation on. This string can optionally use .NET Framework Regular Expressions. The default is ".*", meaning perform this operation on all currently loaded objects.
- usage:string - Which vertex element to remove from the vertex data. If a mesh does not contain vertex data of the given usage, the operation is ignored for that mesh. This argument is required and can be unnamed (i.e. passed in as 'TANGENT' instead of usage:'TANGENT').
- usageIdx:int - Integer to further identify which vertex element to remove. If no usageIdx is specified, this command will match the first valid element that matches the specified usage.
- newUsage:string - D3DDECLUSAGE value. Default is texcoord.
- newUsageIdx:int - Integer value used for the usage index of the new vertex declaration entry. Default value is the first valid index for the specified usage.
Example:
clonevdata usage:TANGENT usageIdx:0 newUsage:TEXCOORD newUsageIdx:1;
Remarks:
The new element is appended to the end of the vertex as well as the vertex declaration.
delvdata Script Command
Description:
Removes vertex elements from the vertex streams associated with the meshes.
Syntax:
delvdata src:string usage:string usageIdx:int;
Where:
- src:string - Specifies which currently loaded objects to perform this operation on. This string can optionally use .NET Framework Regular Expressions. The default is ".*", meaning perform this operation on all currently loaded objects.
- usage:string - The name of the element that is being deleted. The element names are defined in D3DDECLUSAGE (without the D3DDECLUSAGE_ prefix). There is no default value. If the specified parameter is not contained in a given mesh, the operation will not be performed on that mesh. This argument is required and can be unnamed (i.e. passed in as 'TANGENT' instead of usage:'TANGENT').
- usageIdx:int - An integer to further identify which vertex element to remove. If no usageIdx is specified, this command will match the first valid element that matches the specified usage.
Example:
delvdata usage:TANGENT usageIdx:1;
Remarks:
This will remove the specified element from the declaration as well as from the vertices themselves.
flatten Script Command
Description:
Converts all of the meshes in a model into a single non-hierarchical mesh.
Syntax:
flatten dst:string src:string;
Where:
- dst:string - The name of the resulting flattened model. This argument is required and can be unnamed (i.e. passed in as 'nonHierarchicalModel' instead of dst:'nonHierarchicalModel').
- src:string - Specifies which currently loaded objects to perform this operation on. This string can optionally use .NET Framework Regular Expressions. The default is ".*", meaning perform this operation on all currently loaded objects.
Example:
flatten dst:christmasTreeOneMesh src:christmasTreeWithSeparateOrnaments;
Remarks:
This will also remove any skin or animation information from the mesh.
This script command uses D3DXConcatenateMeshes.
genadjacency Script Command
Description:
Generates adjacency data for meshes, replacing existing data if it exists.
Syntax:
genadjacency src:string type:[topological|geometric] epsilon:float;
Where:
- src:string - Specifies which currently loaded objects to perform this operation on. This string can optionally use .NET Framework Regular Expressions. The default is ".*", meaning perform this operation on all currently loaded objects.
- type:[topological|geometric] - Specifies how the adjacency should be created. Either topological (default) or geometric.
- epsilon:float - Epsilon value. Defaults to 10e-6f.
Example:
genadjacency type:topological;
Remarks:
This script command uses ID3DXBaseMesh::ConvertPointRepsToAdjacency for the topological method, and ID3DXBaseMesh::GenerateAdjacency for the geometric method.
genmipmaps Script Command
Description:
Generates mipmaps for the specified textures.
Syntax:
genmipmaps src:string start:int dither:int filter:string;
Where:
- src:string - Specifies which currently loaded objects to perform this operation on. This string can optionally use .NET Framework Regular Expressions. The default is ".*", meaning perform this operation on all currently loaded objects.
- start:int - The zero-based index of the mipmap to use as the source of the generated mipmaps. Default is 0.
- dither:int - Enables or disables dithering during mipmap generation. 0 for false, 1 for true. Default is 1.
- filter:string - The mipmap filter to use when generating the mipmaps. Either None, Point, Linear, Triangle, or Box. Box is the default.
Example:
genmipmaps src:LobbyCube start:0 filter:Linear;
Remarks:
This script command uses D3DXFilterTexture.
gennormals Script Command
Description:
Computes vertex normals for every mesh.
Syntax:
gennormals src:string;
Where:
- src:string - Specifies which currently loaded objects to perform this operation on. This string can optionally use .NET Framework Regular Expressions. The default is ".*", meaning perform this operation on all currently loaded objects.
Example:
gennormals src:tiger;
Remarks:
If no normals are stored in the mesh before this command is called, then a call to the addvdata command must be made first, which will add normal data to the vertex declaration and assign empty values for the normals. Calling this command after adding normals to the vertex declaration will turn the normals into valid values.
This script command uses D3DXComputeNormals.
gentangentframes Script Command
Description:
Computes tangent frames for every mesh.
Syntax:
gentangentframes src:string texIdx:int ortho:string normalizePartials:[0|1] weight:string wrap:string threashPE:float threashSP:float threashNE:float;
Where:
- src:string - Specifies which currently loaded objects to perform this operation on. This string can optionally use .NET Framework Regular Expressions. The default is ".*", meaning perform this operation on all currently loaded objects.
- texIdx:int - Specifies the set of texture coordinates to use for calculating the tangents. 0 specifies the first texture coordinate set, 1 specifies the second texture coordinate set, and so on. The default value is 0.
- ortho:string - Possible values: None, U, V, UV, where None is default. Specifying None generates does not orthonormalize the tangent frame. Specifying U orthonormalizes the tangentframe based on the final computation of the non-orthonormalized tangent vector. Specifying V orthonormalizes the tangentframe based on the final computation of the non-orthonormalized binormal vector. Specifying UV orthonormalizes the tangentframe to the nearest matrix both the non-orthonormalized tangent and binormal vectors.
- normalizePartials:[0|1] - Possible values: 0 or 1, 1 being default. If 0 is specified, it will not normalize partial derivatives with respect to texture coordinates. If not normalized, the scale of the partial derivatives is proportional to the scale of the 3D model divided by the scale of the triangle in (u, v) space. This scale value provides a measure of how much the texture is stretched in a given direction. The resulting vector length is a weighted sum of the lengths of the partial derivatives.
- weight:string - Possible Values: Area, Equal, Default, where Default is default. Specifying Equal computes a unit-length normal vector for each triangle of the input mesh. Specifying Area weights the direction of the computed per-vertex normal or partial derivative vector according to the areas of triangles attached to that vertex. Specifying Default will give weight by angle, which gives weight based on how much angle the triangle covers for the vertex. Specifying Default tends to give results that make more sense.
- wrap:string - Possible Values: None, U, V, UV, where None is default. For details, see Texture Wrapping.
- threshPE:float - Defaults to 0.8f. Specifies the maximum cosine of the angle at which two partial derivatives are deemed to be incompatible with each other. If the dot product of the direction of the two partial derivatives in adjacent triangles is less than or equal to this threshold, then the vertices shared between these triangles will be split.
- threshSP:float - Defaults to 0.5f. Specifies the maximum magnitude of a partial derivative at which a vertex will be deemed singular. As multiple triangles are incident on a point that has nearby tangent frames, but altogether cancel each other out (such as at the top of a sphere), the magnitude of the partial derivative will decrease. If the magnitude is less than or equal to this threshold, then the vertex will be split for every triangle that contains it.
- threshNE:float - Defaults to 0.8f. Similar to threshPE, this specifies the maximum cosine of the angle between two normals that is a threshold beyond which vertices shared between triangles will be split. If the dot product of the two normals is less than the threshold, the shared vertices will be split, forming a hard edge between neighboring triangles. If the dot product is more than the threshold, neighboring triangles will have their normals interpolated.
Example:
gentangentframes texIdx:1 ortho:U weight:Area threshNE:0.5f;
Remarks:
This script command uses D3DXComputeTangentFrameEx.
load Script Command
Description:
Load a mesh or texture file.
Syntax:
load fileName:string srgb:int;
Where:
- fileName:string - Name of a model or texture file. Use the .x extension for model files. Supported texture formats: BMP, DDS, DIB, HDR, JPG, PFM, PNG, PPM, and TGA. This parameter supports wildcards, so *.jpg will load all jpg files in the current working directory, as well as any paths specified with the -m option. This argument is required and can be unnamed (i.e. passed in as 'tiger.x' instead of fileName:'tiger.x').
- srgb:int - Whether or not to treat the image as sRGB. 1 for true, 0 for false. 0 is default.
Example:
load fileName:tiger.x;
Remarks:
If the model file has a hierarchy, the hierarchy will be maintained when loading; if filename contains wildcards, all files matching the file specification are loaded; if filename is not an absolute path, the path is assumed to be relative to the current working directory.
This script command uses D3DXLoadMeshHierarchyFromX if loading a mesh, and D3DXCreateTextureFromFile if loading a texture.
meshes Script Command
Description:
Log information about all models and their meshes into the log file specified in the command line arguments.
Syntax:
meshes src:string;
Where:
- src:string - Specifies which currently loaded objects to perform this operation on. This string can optionally use .NET Framework Regular Expressions. The default is ".*", meaning perform this operation on all currently loaded objects.
Example:
meshes src:tiger;
newtex2d Script Command
Description:
Creates a new 2D texture.
Syntax:
newtex2d dst:string format:string width:int height:int numMips:int src:string;
Where:
- dst:string - Name of the new texture. This argument is required and can be unnamed (i.e. passed in as 'newTex' instead of dst:'newTex').
- format:string - Any of the following formats: A16B16G16R16, A16B16G16R16F, A1R5G5B5, A2B10G10R10, A2R10G10B10, A2W10V10U10, A32B32G32R32F, A4L4, A4R4G4B4, A8, A8B8G8R8, A8L8, A8R3G3B2, A8R8G8B8, DXT1, DXT2, DXT3, DXT4, DXT5, G16R16, G16R16F, G32R32F, L16, L8, R16F, R32F, R3G3B2, R5G6B5, R8G8B8, X1R5G5B5, X4R4G4B4, X8B8G8R8, or X8R8G8B8. See D3DFORMAT for more information about each format. Required argument.
- width:int - Width of the texture, in pixels. Required argument.
- height:int - Height of the texture, in pixels. Required argument.
- numMips:int - The number of mipmaps to allocate within the texture. The default is 0 meaning to create as many mipmaps as necessary for the width and height.
- src:string - Name of a source texture used to initialize the new texture. This must already be loaded. The default is 0 meaning no source texture is used.
Example:
newtex2d dst:newLobby format:A16B16G16R16 width:500 height:500;
Remarks:
This script command uses IDirect3DDevice9::CreateTexture.
newtex3d Script Command
Description:
Creates a new cube texture.
Syntax:
newtex3d dst:string format:string width:int height:int depth:int numMips:int src:string;
Where:
- dst:string - Name of the new texture. This argument is required and can be unnamed (i.e. passed in as 'newTex' instead of dst:'newTex').
- format:string - Any of the following formats: A16B16G16R16, A16B16G16R16F, A1R5G5B5, A2B10G10R10, A2R10G10B10, A2W10V10U10, A32B32G32R32F, A4L4, A4R4G4B4, A8, A8B8G8R8, A8L8, A8R3G3B2, A8R8G8B8, DXT1, DXT2, DXT3, DXT4, DXT5, G16R16, G16R16F, G32R32F, L16, L8, R16F, R32F, R3G3B2, R5G6B5, R8G8B8, X1R5G5B5, X4R4G4B4, X8B8G8R8, or X8R8G8B8. See D3DFORMAT for more information about each format. Required argument.
- width:int - The width of the texture, in pixels. Required argument.
- height:int - The height of the texture, in pixels. Required argument.
- depth:int - The depth of the texture. Required argument.
- numMips:int - The number of mipmaps to allocate within the texture. The default is 0 meaning to create as many mipmaps as necessary for the size.
- src:string - Name of a source texture used to initialize the new texture. This must already be loaded. The default is 0 meaning no source texture is used.
Example:
newtex3d dst:newLobby3D format:A16B16G16R16F width:500 height:500;
Remarks:
This script command uses IDirect3DDevice9::CreateVolumeTexture.
newtexcube Script Command
Description:
Creates a new cube texture.
Syntax:
newtexcube dst:string format:string size:int numMips:int srcXP:string srcXM:string srcYP:string srcYM:string srcZP:string srcZM:string;
Where:
- dst:string - Name of the new texture. This argument is required and can be unnamed (i.e. passed in as 'newTex' instead of dst:'newTex').
- format:string - Any of the following formats: A16B16G16R16, A16B16G16R16F, A1R5G5B5, A2B10G10R10, A2R10G10B10, A2W10V10U10, A32B32G32R32F, A4L4, A4R4G4B4, A8, A8B8G8R8, A8L8, A8R3G3B2, A8R8G8B8, DXT1, DXT2, DXT3, DXT4, DXT5, G16R16, G16R16F, G32R32F, L16, L8, R16F, R32F, R3G3B2, R5G6B5, R8G8B8, X1R5G5B5, X4R4G4B4, X8B8G8R8, or X8R8G8B8. See D3DFORMAT for more information about each format. Required argument.
- size:int - Size of one edge of the cube texture, in pixels. Required argument.
- numMips:int - The number of mipmaps to allocate within the texture. The default is 0 meaning to create as many mipmaps as necessary for the size.
- srcXP:string - Name of a source texture used to initialize the positive x face. This must already be loaded into memory. The default is 0 meaning no source texture is used.
- srcXM:string Name of a source texture used to initialize the negative x face. This must already be loaded into memory. The default is 0 meaning no source texture is used.
- srcYP:string - Name of a source texture used to initialize the positive y face. This must already be loaded into memory. The default is 0 meaning no source texture is used.
- srcYM:string - Name of a source texture used to initialize the negative y face. This must already be loaded into memory. The default is 0 meaning no source texture is used.
- srcZP:string - Name of a source texture used to initialize the positive z face. This must already be loaded into memory. The default is 0 meaning no source texture is used.
- srcZM:string - Name of a source texture used to initialize the negative z face. This must already be loaded into memory. The default is 0 meaning no source texture is used.
Example:
newtexcube dst:newEnvironmentMap format:A16B16R16F size:512;
Remarks:
This script command uses IDirect3DDevice9::CreateCubeTexture.
optimize Script Command
Description:
Optimizes and welds vertices the meshes.
Syntax:
optimize src:string splits:int noCache:int weldAll:[none|epsilon|all] e:float P:float W:float N:float T:float B:float UV:float D:float S:float PS:float F:float;
Where:
- src:string - Specifies which currently loaded objects to perform this operation on. This string can optionally use .NET Framework Regular Expressions. The default is ".*", meaning perform this operation on all currently loaded objects.
- splits:int - Whether or not vertices shared between attribute groups can be split 1 means no splits allowed, 0 (default) means splits are allowed.
- noCache:int - Whether or not the optimization should use a vertex cache size based on information from the current device. The default is 1, meaning that the device vertex cache should be ignored.
- weldAll:[none|epsilon|all] - Epsilon is the default value. If none is specified, no vertices will be welded. If epsilon is specified, it will weld vertices only if they are within the specified epsilon values. If all is specified, it will weld vertices reguardless of epsilon values.
- e:float - Epsilon value for the welding algorithm to use across all components. Default value: 10e-6f. If this is specified, all other epsilon values are assigned to this value.
- P:float - Position epsilon value. The welding algorithm will check this value before all other epsilon values when determining if two vertices need to be welded.
- W:float - Blend Weights epsilon value.
- N:float - Normals epsilon value.
- T:float - Tangents epsilon value.
- B:float - Binormals (or Bitangents) epsilon value.
- UV:float - Texture Coordinates epsilon value.
- D:float - Diffuse Colors epsilon value.
- S:float - Specular Colors epsilon value.
- PS:float - Point Sizes epsilon value.
- F:float - Tessellation Factors epsilon value.
Example:
optimize e:10e-9f;
Remarks:
This optimization allows the meshes to be more optimal for performance. The primary optimization that this command does is vertex welding, which optimizes the mesh by removing duplicate vertices.
This script command uses D3DXWeldVertices and ID3DXMesh::Optimize.
reformat Script Command
Description:
Changes the format of the specified textures.
Syntax:
reformat src:string format:string;
Where:
- src:string - Specifies which currently loaded objects to perform this operation on. This string can optionally use .NET Framework Regular Expressions. The default is ".*", meaning perform this operation on all currently loaded objects.
- format:string - Any of the following formats: A16B16G16R16, A16B16G16R16F, A1R5G5B5, A2B10G10R10, A2R10G10B10, A2W10V10U10, A32B32G32R32F, A4L4, A4R4G4B4, A8, A8B8G8R8, A8L8, A8R3G3B2, A8R8G8B8, DXT1, DXT2, DXT3, DXT4, DXT5, G16R16, G16R16F, G32R32F, L16, L8, R16F, R32F, R3G3B2, R5G6B5, R8G8B8, X1R5G5B5, X4R4G4B4, X8B8G8R8, or X8R8G8B8. See D3DFORMAT for more information about each format. This argument is required and can be unnamed (i.e. passed in as 'A8L8' instead of format:'A8L8').
Example:
reformat format:A4R4G4B4;
Remarks:
This script command uses D3DXLoadSurfaceFromSurface.
reset Script Command
Description:
Resets as if DXOps has been restarted. Equivalent to calling unload on all objects.
Syntax:
reset;
resize Script Command
Description:
Resizes a texture.
Syntax:
resize src:string width:int height:int depth:int dither:int filter:string;
Where:
- src:string - Specifies which currently loaded objects to perform this operation on. This string can optionally use .NET Framework Regular Expressions. The default is ".*", meaning perform this operation on all currently loaded objects.
- width:int - The width of the texture, in pixels. Required argument.
- height:int - The height of the texture, in pixels. Required argument.
- depth:int - The depth of the texture.
- dither:int - Enables or disables dithering during mipmap generation. 0 for false, 1 for true. Default is 1.
- filter:string - The mipmap filter to use when generating the mipmaps. Either None, Point, Linear, Triangle, or Box. Box is the default.
Example:
resize width:200 height:200;
Remarks:
This script command uses D3DXLoadSurfaceFromSurface.
save Script Command
Description:
Saves the models or textures to a file.
Syntax:
save src:string fileName:string xFileType:string;
Where:
- src:string - Specifies which currently loaded objects to save. This string can optionally use .NET Framework Regular Expressions, but the expressions must resolve to one item. The default is ".*", meaning perform this operation on all currently loaded items.
- fileName:string - Name of destination output file. This argument is required and can be unnamed (i.e. passed in as 'tiger.x' instead of fileName:'tiger.x').
- xFileType:string - If saving to an x file, this is used to determine the type of x file. Can be either text (default), binary, or compressed. Text is the recommended format.
Example:
save src:tiger fileName:tiger_modded.x xFileType:text;
Remarks:
This script command uses D3DXSaveMeshHierarchyToFile if saving a model.
shade Script Command
Description:
Calls a user-defined HLSL shader to modify a texture.
Syntax:
shade src:string shader:string fileName:string ...parameters...;
Where:
- src:string - The name of the target texture. This string can optionally use .NET Framework Regular Expressions. The default is ".*", meaning perform this operation on all currently loaded textures.
- shader:string - Name of the shader to use. Default value is main.
- fileName:string - Name of the text file that contains the HLSL shader. This argument is required and can be unnamed (i.e. passed in as 'texShader.psh' instead of fileName:'texShader.psh').
- ...parameters... - corresponds to the names of the parameters specified in the HLSL shader file. See teh Passing Parameters Into Shaders section below.
Passing Parameters Into Shaders
The shade script command allows you to initialize parameters in your HLSL shader. Doing so follows the basic syntax <name of parameter>:string. The string will be used initialize the specified parameter and the format of that string will depend on the data type of parameter that you are initializing.
For integers, floating point numbers, and matrices, the values are passed in as comma-delimited lists of numbers. For example:
float pi;
float3 viewVec;
matrix4x4 viewMat;
Would be initialized in the shade command by:
pi:'3.14' viewVec:'0,1,0' viewMat:'1,0,0,0,0,1,0,0,0,0,1,0,0,0,0,1'
Textures are passed into the shader by the name of their sampler. For example:
textureCUBE TableClothTex;
samplerCUBE TableClothSamp = sampler_state
{
Texture = (TableClothTex);
MinFilter = Linear;
MagFilter = Linear;
};
Would be initialized in the shade command by:
TableClothSamp:'TabCloth'
Where TabCloth is a texture loaded into DXOps before the shade command was called. Below is an example usage of the shade script command that will create a texture that blends from white to black in the y direction.
If the HLSL file defines a sampler parameter with the name "Target", then the shade script command will automatically fill that parameter with the target texture. See the Using the Shade Script Command example.
HLSL Function Requirements
The function definitions for these HLSL texture shaders follow a syntax slightly different from vertex and pixel shaders. There can be three types of texture shader functions - one for 2D textures, one for 3D textures, and one for cube textures - each of which follows this convention:
For 2D textures:
float4 main(float2 UV : TEXCOORD0, float2 PixelSize : TEXCOORD1) : COLOR
Where UVs are 0 to 1
For 3D textures:
float4 main(float3 UVW : TEXCOORD0, float3 PixelSize : TEXCOORD1) : COLOR
Where UVWs are 0 to 1.
For cube textures:
float4 main(float3 UVW : TEXCOORD0, float3 PixelSize : TEXCOORD1) : COLOR
Where UVWs are -1 to 1.
PixelSize will have a zero on one of the axes. This will indicate which axis of the cube you are currently shading. Look to the sign of the corresponding axis in the UVW variable to determine if you are working on the positive or negative face along that axis. See the Using the Shade Script Command example.
Example:
newtex2d dst:'test' format:A8R8G8B8 width:64 height:64 mips:0;
shade fileName:'ramp.psh' top:'1,1,1' bottom:'0,0,0';
save fileName:'t-ShadeTex2.dds';
Where ramp.psh is:
float3 top;
float3 bottom;
float4 main(float2 UV : TEXCOORD0, float2 PixelSize : TEXCOORD1) : COLOR
{
return float4( lerp(bottom, top, UV.yyy ), 1);
}
This example will generate an image that looks like this:
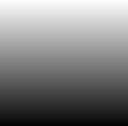
Remarks:
This will not work on DXT compressed textures. If you have a DXT compress texture and wish to run the shade command on it, try reformatting the texture to a format that is valid as a render target and then reformatting it back to the desired format afterwards.
reformat 'A16B16G16R16F';
shade 'RedXGreenYPlusTarget-cube.psh';
reformat 'Dxt1';
This script command uses D3DXFillTextureTX.
slice2d Script Command
Description:
Creates a new 2D texture from an existing texture surface.
Syntax:
slice2d dst:string src:string volume:int face:string mipMap:int;
Where:
- dst:string - The name of the texture to be generated. This argument is required. A regular expression may be used for this argument as long as it resolves to one name. This argument is required and can be unnamed (i.e. passed in as 'resizedTex' instead of dst:'resizedTex').
- src:string - The source image from which the surface will be extracted. This must already be loaded. Required argument.
- volume:int - If extracting from a volume texture, this specifies the surface to be extracted. This uses zero-based indexing. The default value is 0.
- face:string - If extracting from a cube texture, this specifies the face to be extracted. This can either be +x, -x, +y, -y, +z, or -z. The default is +x.
- mipMap:int - If extracting from a mipmap texture, this specifies the zero-based index into the mipmap. The default value is 0.
Example:
slice2d dst:bliss2 src:bliss;
Remarks:
This script command uses D3DXLoadSurfaceFromSurface.
splice2d Script Command
Description:
Inserts a surface into an existing texture.
Syntax:
splice2d dst:string src:string volume:int face:string mipMap:int;
Where:
- dst:string - The name of the destination texture. A regular expression may be specified here for multiple destinations. This argument is required and can be unnamed (i.e. passed in as 'destTex' instead of dst:'destTex').
- src:string - The name of the source texture. This must already be loaded into memory. Required argument.
- volume:int - If pasting into a volume texture, this specifies the destination surface using zero-based indexing. The default value is 0.
- face:string - If pasting into a cube texture, this specifies the destination face. This can either be +x, -x, +y, -y, +z, or -z. The default is +x.
- mipMap:int - If pasting into a mipmap texture, this specifies the destination surface using zero-based indexing. The default value is 0.
Example:
splice2d dst:bliss src:azul;
Remarks:
This script command uses D3DXLoadSurfaceFromSurface.
stripify Script Command
Description:
Attempts to optimize meshes by ordering their triangles into strips.
Syntax:
stripify src:string splits:[0|1];
Where:
- src:string - Specifies which currently loaded objects to perform this operation on. This string can optionally use .NET Framework Regular Expressions. The default is ".*", meaning perform this operation on all currently loaded objects.
- splits:[0|1] - Whether or not the operation will be allowed to introduce duplicate vertices. It is recommended to allow the algorithm to introduce duplicates because otherwise the resulting triangle strips could render inefficiently. Default value is 1.
Example:
stripify src:tiger;
Remarks:
Does the following:
- Minimizes the number of attribute groups by performing an attribute sort.
- Reorders the mesh faces to maximize strip length.
- Converts every mesh subset of every mesh to a single triangle strip.
This script command uses ID3DXMesh::Optimize (with D3DXMESHOPT_STRIPREORDER).
textures Script Command
Description:
Log information about all meshes into the log file specified in the command line arguments.
Syntax:
textures src:string;
Where:
- src:string - Specifies which currently loaded objects to perform this operation on. This string can optionally use .NET Framework Regular Expressions. The default is ".*", meaning perform this operation on all currently loaded objects.
unload Script Command
Description:
Removes the specified texture(s) or model(s) from DXOps.
Syntax:
unload src:string type:string;
Where:
- src:string - Specifies which currently loaded objects to perform this operation on. This string can optionally use .NET Framework Regular Expressions. The default is ".*", meaning perform this operation on all currently loaded objects. This argument is required and can be unnamed (i.e. passed in as 'tiger' instead of src:'tiger').
- type:string - Possible values: models or textures. Specifies the type of object that you wish to unload.
Example:
unload tiger type:models;
uvatlas Script Command
Description:
Partitions and packs a uvatlas for the meshes.
Syntax:
uvatlas src:string maxCharts:int stretch:float gutter:int width:int height:int;
Where:
- src:string - Specifies which currently loaded objects to perform this operation on. This string can optionally use .NET Framework Regular Expressions. The default is ".*", meaning perform this operation on all currently loaded objects.
- maxCharts:int - The maximum number of charts to partition the mesh into. Use 0 to tell D3DX that the atlas should be parameterized based on stretch.
- stretch:float - The amount of stretching allowed. 0 means no stretching is allowed, 1 means any amount of stretching can be used.
- gutter:int - The minimum distance, in texels, between two charts on the atlas. The gutter is always scaled by the width; so, if a gutter of 2.5 is used on a 512x512 texture, then the minimum distance between two charts is 2.5 / 512.0 texels.
- width:int - Texture width.
- height:int - Texture height.
Example:
uvatlas maxCharts:0 stretch:0.5 gutter:2.5 width:512 height:512;
Remarks:
This script command uses D3DXUVAtlasCreate.
validate Script Command
Description:
Validates meshes and sends warnings to the log if there are any.
Syntax:
validate src:string;
Where:
- src:string - Specifies which currently loaded objects to perform this operation on. This string can optionally use .NET Framework Regular Expressions. The default is ".*", meaning perform this operation on all currently loaded objects.
Example:
validate src:tiger;
Remarks:
Checks the following data:
- Backfacing - triangles that share the same vertex indices but have face normals pointing in opposite directions (back-facing triangles).
- Bowties - If a vertex is the apex of two triangle fans (a bowtie) and mesh operations will affect one of the fans. Bowties can cause problems for operations such as mesh simplification that remove vertices.
This script command uses D3DXValidMesh.
See Also
DirectX Content Tools, DirectX Ops (dxops.exe)