Declaring Class Instances
Class instances are usually called objects, but let’s keep calling them instances for a little while longer. Creating them works like this:
Dim thing As CThing
Set thing = New CThing
thing.Title = “Things Fall Apart”
thing.Count = 6
You can’t just declare a variable and start partying on it. You have to create a New one first (often with Set). Bear with me if you think you already know why.
The reason you have to use New is because with class instances, the variable isn’t the same as the instance. Figure 3-1 shows the difference. The variable is your only means of communicating with the instance, but it’s not the same thing.
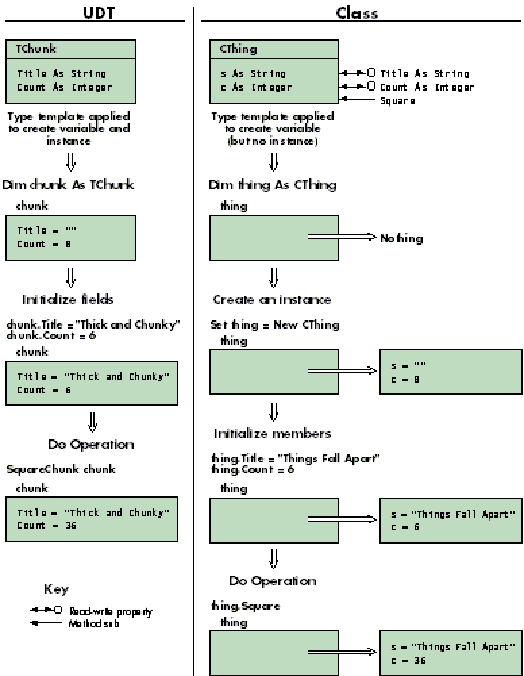
Figure 3-1. User-defined types versus classes.
Let’s look at an example that illustrates the difference:
Dim chunkJoe As TChunk, chunkSue As TChunk
Dim thingJoe As CThing, thingSue As CThing
Like all Visual Basic variables, these are automatically initialized. The TChunk variables have their strings initialized to vbNullString and their integers to 0. The CThing variable doesn’t have an instance yet, so the instance can’t be initialized, but the variable is initialized to Nothing. To make them do something useful, you can assign properties as follows:
chunkJoe.Title = “Call me Joe”
chunkSue = chunkJoe
Set thingJoe = New CThing
thingJoe.Title = “Call me Joe”
Set thingSue = thingJoe
It might seem that you’ve created four objects and set them all to the same value. If you test in the Immediate window, the results indicate that everything is the same:
? chunkJoe.Title, chunkSue.Title
Call me Joe Call me Joe
? thingJoe.Title, thingSue.Title
Call me Joe Call me Joe
This is an illusion, as you can see if you change Title:
chunkSue.Title = “My name is Sue”
thingSue.Title = “My name is Sue”
Print out the values:
? chunkJoe.Title, chunkSue.Title
Call me Joe My name is Sue
? thingJoe.Title, thingSue.Title
My name is Sue My name is Sue
Each TChunk variable contains its instances, so changing chunkSue doesn’t affect chunkJoe. But the thingJoe and thingSue variables both reference the same instance. Changing one changes the other because there isn’t any other. If you want another, you have to create it with the New operator:
Set thingJoe = New CThing
thingJoe.Title = “Call me Joe”
Set thingSue = New CThing
thingSue.Title = “Call me Sue”
This difference between instances of classes and the instances of other types is so fundamental that we generally use different names. With classes, variables are called object variables and instances are called objects. With other types, the term variable usually refers both to the variable and the instance, because they are the same. I’ll switch to this more standard terminology from here on out.
This certainly isn’t the only way a language can declare, create, initialize, and use objects. But it is the most efficient way for a language to manage objects if you don’t have pointers, which is why Java looks to me more like Visual Basic than like C++.