Test Drive
When I write a new class, I start by creating a test form that exercises the class. I put a command button and either a large label or a TextBox control on the form. Before I start writing the class itself, I declare an object of the proposed class in the command buttons Click event procedure and write some code that uses the properties and methods of the class, outputting any status information to the label or the text box. Normally, I name the test program using the name preceded by a T, for example, TDRIVE.FRM to test the CDrive class in DRIVE.CLS. In this case, however, I combined drive testing with testing of other information classes and forms, including an About form. The actual name of the test project is ALLABOUT.FRM in ALLABOUT.VBG. You can see it, with output from CDrive, in Figure 3-2.
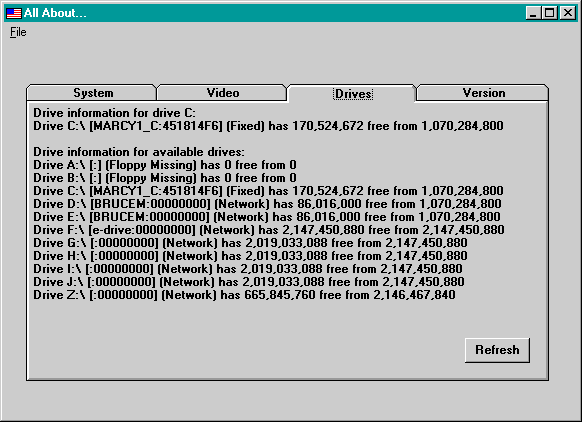
Figure 3-2. The Drives tab of the All About program.
To examine the class, lets start by declaring three CDrive object variables:
Dim driveDat As New CDrive
Dim driveCur As New CDrive
Dim driveNet As New CDrive
At this point, the drive objects have no meaning; they arent yet connected with any physical disk drive. You must somehow connect each Basic drive object to a real-world drive object.
The modern way of specifying a drive is with a root paththe standard system introduced by the Win32 API. Lettered drives have root paths in the form D:\, and network drives that are not associated with letters have root paths in the form \\server\share\. Notice that root paths always end in a backslash. Our CDrive class will have a Root property as the means of specifying a drive:
driveDat.Root = a:\ Set to lettered drive
driveCur.Root = sEmpty Set to current drive
driveNet.Root = \\brucem1\root\ Set to network drive
Back in the ancient days of MS-DOS, drives were sometimes represented by the numbers 0 through 26 (with 0 representing the current drive) and sometimes by the numbers 0 through 25 (with 0 representing drive A). You needed to read the documentation carefully to see which system to use for any MS-DOS function call. One of the reasons for using a class is to eliminate such inconsistencies. The CDrive class recognizes numeric Root property values, with the number 0 representing the current drive and the numbers 1 through 26 representing local drives.
You can initialize a drive as follows:
driveDat.Root = 1 Set to A:
driveCur.Root = 0 Set to current drive
Of course, in Basic a class can have a default property, and the obvious one for the CDrive class is Root:
driveDat = 1 Set to A:
driveCur = 0 Set to current drive
driveDat = a:\ Set to lettered drive
driveCur = sEmpty Set to current drive
driveNet = \\brucem1\root\ Set to network drive
If you dont like any of those, you dont have to set anything. By default, the Root property will be sEmpty and youll get the default drive.
Once the drive is initialized, you can read its Root property (which has been converted to the root path format) or its Number property. The Number property is 0 for network drives:
Assume that current drive is C:
Debug.Print driveDat.Number Prints 1
Debug.Print driveCur.Number Prints 3
Debug.Print driveNet.Number Prints 0
Debug.Print driveDat.Root Prints A:\
Debug.Print driveCur.Root Prints C:\
Debug.Print driveNet.Root Prints \\BRUCEM1\ROOT\
Of course, what you really want is the drive data in the form of read-only properties FreeBytes, TotalBytes, Label, Serial, and KindStr. You can use them this way:
Const sBFormat = #,###,###,##0
With driveCur
s = s & Drive & .Root & [ & .Label & : & _
.Serial & ] ( & .KindStr & ) has & _
Format$(.FreeBytes, sBFormat) & free from & _
Format$(.TotalBytes, sBFormat) & sCrLf
End With
This code displays information in the following format:
Drive C:\ [BRUCEM1:1F81754F](Fixed) has 3 bytes free from 1,058,767,000
The CDrive class is designed with the assumption that drive objects dont change their properties. In real life, of course, drives sometimes do change. You might remove a 14.4 megabyte disk from your A drive and insert a 7.2 megabyte disk. You might disconnect a large network drive from your O drive and connect a small one. CD-ROM technology might change while your program is running. You can make sure that a drive object reinitializes its state from the drive it represents by setting the object variable to a new drive object or by assigning the Root property (even if you reassign the current value).