. Note that it's necessary not only o pass the @id parameter
from which you wish to retrieve records above, but also to specify a descending
option to the ORDER BY clause that puts the rows into the temp table. From
there, it's just a matter of now retrieving them out in the default, ascending
order.
Here's the VB code that lets the user browse backward and forward in the
result set using VCR buttons and a list box (see Figure
1). This subroutine gets pages based on a direction
argument used in the call (next or previous). We save public variables for
the maximum and minimum primary key values in the current recordset as references
for navigating through the virtual recordset (the entire jobs table of the
pubs database). 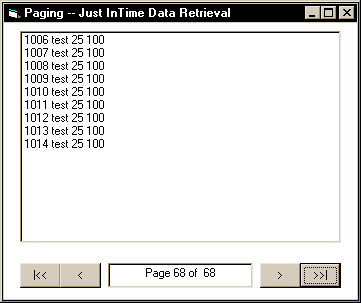
Private Sub GetPage(ByVal Direction As Integer)
Dim strSQL As String
Dim strResults As String
Dim adoCmd As adodb.Command
Dim adoRS As adodb.Recordset
Dim adoParam As adodb.Parameter
Dim adoField As adodb.Field
Dim intInput As Long
Set adoRS = New adodb.Recordset
'Open a recordset to get a rowcount for the jobs table.
'This is a faster way to get a rowcount than "Select
'count(*) from jobs".
With adoRS
.ActiveConnection = adoConn
.Source = "select rows from sysindexes where id = _
object_id('jobs') and indid < 2"
.Open
End With
plngRows = adoRS(0)
adoRS.Close
Set adoRS = Nothing
'Get the number of pages based on the number of rows
'and the rows per page setting.
plngPages = CLng(plngRows / plngRowsPerPage)
'Correct the number of pages for the rounding
'of the CInt function.
If (plngRows / plngRowsPerPage) > plngPages Then
plngPages = plngPages + 1
End If
'If we're on the last page, don't try to move next.
'If we're on the first page, don't move to previous.
If (plngPage = plngPages And Direction = MOVE_NEXT) _
Or (plngPage = 1 And Direction = MOVE_PREV) Then
Exit Sub
End If
|
Here's the event procedure to move to the very first page:
Private Sub cmdFirstPage_Click(Index As Integer)
'Move to the first "page" by setting the starting
'point primary key value to 0 and the page # to 1.
plngMax = 0
plngPage = 1
GetPage MOVE_NEXT
'Set the page number text box value.
pstrPages = "Page " & plngPage & " of " & _
Str(plngPages)
txtPageNo = pstrPages
End Sub
|
Here's the event procedure code for moving to the next page (the code for
moving backwards is similar):
Private Sub cmdNextPage_Click(Index As Integer)
'Move to the next "page".
GetPage MOVE_NEXT
'If we're on the last page, then don't change the page
'number text box value.
If plngPage = plngPages Then
Exit Sub
Else
plngPage = plngPage + 1
pstrPages = "Page " & plngPage & " of " & _
Str(plngPages)
txtPageNo = pstrPages
End If
End Sub
|
The key to going to the last page is to set the starting point primary key
value to the appropriate value based on the rows per page setting, and then
calculating the number of pages. You can view the source code in the accompanying
Download file.
Download sample code for this article here.
Bob Pfeiff , an MCSD and SQL
Server MVP, works for Spectrum Technology Group as a senior consultant and
project manager in McLean, VA. Bob authored a chapter in SQL Server 6.5 Unleashed (third edition) and
is writing a chapter for the forthcoming SQL Server 7.0 version. bobp@spectrumtech.com.
Al Noel, an MCSD, MCSE, and MCT, works for Spectrum as a consultant. Al
has been in the computer business for 18 years, including a year at the
AI lab at MIT as a visiting scientist. anoel@netcom.com.