January 1999
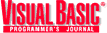 |
|
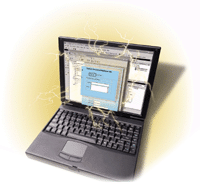 More Web Power With VB6 and IE5
Use Visual Basic 6 to Make Internet Explorer 5's
New Features Work for You
by Charles Crawford Caison, Jr.
Reprinted with permission from Visual Basic Programmer's Journal, Jan 1999, Volume 9, Issue 1, Copyright 1999, Fawcette Technical Publications, Palo Alto, CA, USA. To subscribe, call 1-800-848-5523, 650-833-7100, visit www.vbpj.com, or visit The Development Exchange.
Product changes and improvements aren't always on the surface. Internet
Explorer 5 (IE5) looks much like its predecessor, IE4, because most of
the changes are "under the hood": They extend and enhance IE5's programmability, improve performance, enhance Web applications, and make Dynamic HTML (DHTML) application development easier. To fully exploit IE5's potential, you can leverage some new features of VB6: IIS Applications and DHTML Applications.
What you need:
Internet Explorer 5.0 Developer Preview Release for Windows 95 and NT
Visual Basic 6.0
|
|
In this article, I'll detail some crucial new IE5 features and explain how to use them from within Visual Basic 6. Although you'll spend the majority of your time inside VB6 to build your Web application, you'll occasionally modify your Web page directly using another editor. I'll use the fictitious TravelCorp Travel Agency Web site to demonstrate the new IE5 features. Using the TravelCorp Web site, users can plan vacations, view the cost of a vacation, and even review company stock information. The TravelCorp Web site application is a VB6 DHTML Application (see Figure 1).
The IE and VB Connection
VB6's two important new project types enable you to harness the power of IE5. The new IIS Application project type empowers the VB6 developer with server-side processing similar to Active Server Pages (ASP). The new DHTML Application project type enables Internet applications to perform sophisticated processing within the browser. Also, installing IE5 automatically upgrades the Internet control. VB developers can add the control to customize applications to use the new IE5 enhancements.
The IIS Application project type focuses primarily on server-side processing, and doesn't provide direct support for DHTML client-side processing. However, IE5 has some impact on IIS Applications. If you're familiar with creating Web pages and writing scripts, IIS Applications can be even more powerful and flexible than DHTML Applications. The downside is that you must create DHTML from within an IIS Application by hand or with another DHTML code generator. Consider that IIS Applications run code on the server side and generate HTML based on the results of that processing. If you realize that the output can be any text, including DHTML and client-side script, you understand IIS Applications' real power. If your server-side processing can control exactly what DHTML script gets sent to the browser, and your browser can exploit DHTML when it gets there, you have the ingredients for an incredibly flexible system that executes code on both the client and server sides. You can use all IE5 features within a page from an IIS Application, but you would need to write DHTML code with little DHTML support from the IIS Application.
DHTML Applications can help Visual Basic developers make the transition into DHTML programming. Using the familiar and powerful Visual Basic Integrated Development Environment (IDE) and language, you can write to DHTML Application events just as you've written to client/server application events.
To create a VB6 DHTML Application, select DHTML Application from the New Project dialog box that appears when you start VB6. (It also appears when you select the File | New Project menu item.) When the DHTML Application starts, your project includes a single code module and a single DHTML designer file. The VB6 IDE includes a number of items when you create a DHTML Application (see Figure 2). The DHTML designer enables you to add text, tables, images, and colors to the page. On the left, an object treeview displays the items on your page and the hierarchical relationships between them. Using the toolbar across the top of the DHTML designer, you can create hyperlinks, set font style and alignment properties, set the z-order (front-to-back) of page objects, and add tables.
The real power of DHTML Applications lies in the fact that you can associate events, such as mouseover and click, with page objects, and you can write code to those events. Most objects have an ID property. When you assign a value to an object's ID, it inherits events and becomes available to VB code modules. The DHTML designer doesn't help create Cascading Style Sheets (CSS), and it doesn't provide a mechanism for adding a reference to an existing CSS. CSS files store style information for page elements, such as <P>, <DIV>, and <H1> tags. Any text within the tags is rendered in the way that the CSS defines, including font size, font color, and background color. Alternatively, you can specify style information in code within a DHTML Application. The sample application for this article includes style information in both ways. You must use an external editor to create a CSS and link the CSS to your DHTML page.
A Little Behavior Modification
DHTML Behaviors (also known simply as Behaviors) are one of IE5's most exciting and innovative features. Using IE5 Behaviors, you can link a separate script file into your HTML file that controls how various page objects function. Behaviors can enhance intrinsic HTML controls, enabling them to perform any function underlying script code can support. In other words, you can turn moderately useful HTML elements into powerful page objects that rival ActiveX controls and Java applets in functionality. For example, you can use a Behavior to make a standard HTML textbox behave like a ticker-tape control that continuously scrolls text. You can make an HTML table display a working calendar, or you can turn an HTML label into a hyperlink control.
Just as you can separate content from presentation with style information, you can separate content from programming logic using IE5. This helps break the application into manageable pieces. DHTML Applications support this intrinsically, without IE5. However, code in a DHTML Application isn't automatically reusable, as Behaviors are.
A Behavior is simply a plain text file that includes script. The file has an SCT extension and the script in the file references and manipulates objects on the Web page. This new architecture brings the benefits of encapsulation and code reuse to the world of DHTML. Doing so results in a cleaner, more manageable page, and enables you to reuse the same code across multiple pages.
Behaviors, by definition, are scripts. Visual Basic isn't designed to create or edit scripts. You can use Visual InterDev, Visual Notepad, or another editor to create the Behaviors. After you create a Behavior, you can reference it from within a Web page itself or from within your VB6 DHTML application. To make this work, add an element to a DHTML page, then apply a Behavior to the page element. Because the code exists in a separate, reusable file, you don't need to add script code to the DHTML page. You can apply a Behavior to a page element in four different ways. Three of the methods require modifying the Web page directly, through an external editor. The fourth enables you to link the Behavior programmatically, from within a procedure in your DHTML Application.
The first way to apply a Behavior: Include it in <STYLE> tags on the Web page. The link identifies the Behavior by providing the name and path to the external Behavior SCT file:
<STYLE>
.StockTicker { behavior : url(StockTicker.sct)}
</STYLE>
<INPUT TYPE=TEXT ID=txtMyStockTicker Class=StockTicker>
The second way: Associate a Behavior with a page element as part of an HTML element selector. Anytime you use the tag, it uses the corresponding Behavior. In this code, txtMyStockTicker inherits the StockTicker Behavior:
<STYLE>
INPUT { behavior : url(StockTicker.sct)}
</STYLE>
<INPUT TYPE=TEXT ID=txtMyStockTicker>
As a third way, you can associate a Behavior with a page element using the Style property:
<INPUT TYPE=TEXT ID=txtMyStockTicker STYLE="
behavior:url(StockTicker.sct)">
In the fourth way, you associate a behavior with a page element in code:
TxtMyStockTicker.style.behavior = _
"behavior:url(StockTicker.sct)"
Behaviors consist mainly of script, and possibly XML tags that define the Behavior and, in some cases, expose a property. The new attachEvent method runs inside the Behavior script file itself and enables a Behavior scriptlet to listen to events fired on the element and handle the events for that element. For example, using attachEvent, you can forward a click event on a textbox to a Behavior and handle the click event there. This code shows the attachEvent procedure calls made within a Behavior file:
Object.attachEvent("onfocus", doFocus)
Object.attachEvent("onblur", doBlur)
When the onFocus event fires on the object in IE5, the scriptlet automatically steps in and runs its own doFocus function the Behavior script file defines. Within these functions, the contents of the text files are modified to fit a particular format.
A Web page can refer to a Behavior located on another server or in another domain. This allows flexibility, but do this with caution because the Behavior might change or move without prior notice, leading to undesirable behavior on the page. Consider using Behaviors from within a trusted domain so the page always works as expected.
IE5's new persistence technology provides a rich XML-based way to persist data across pages. Any element on a page, such as a textbox, can maintain its current data, even when users leave a page and return later. While developers can do this in a limited way with cookies, this new method eliminates the cookie 4K limit.
Several of the five built-in DHTML Behaviors included with IE5 enable you to instruct the browser to preserve Web page information. For example, with a page that has an expanding table of contents, you can expand the listing, find the link you're looking for, and follow it somewhere else; when you return to it later, the outline is expanded just as you left it. You can persist form data, styles, state, and script variables in four ways (see Table 1).
Assign Property Values at Run Time Without Code
The new setExpression function adds an expressionany valid codeto an object's property. You can use the setExpression function in multiple ways. Two common uses: to dynamically manipulate the position or size of a page element, and to dynamically manipulate the text or HTML display of a page element. For example, you can use setExpression to make a textbox consume the entire width of the available client area in the browser. The textbox always fits exactly into the window, regardless of the browser windows' width.
You can also use setExpression to dynamically set the text or innerHTML properties of an element on a page. You can alter the HTML of a page after the browser loads it, and without making a trip to the server. Use formatting tags, such as <input>, <b>, and <img>, to render new HTML. The setExpression method takes three input parameters. The first parameter to the setExpression method, which is required, is the name of the property to which the expression will be added. The second parameter, also required, is any valid code that will be added to the property. The third parameter, which is optional, names the language of the code in the second parameter. Valid values include Jscript, JavaScript, and VBScript. Here's an example of how to use setExpression:
H1.setExpression "innerHTML", _
"txtNewValue.value", "
Note that the innerHTML property of the element named H1 is changed. The changes come from the value property of the txtNewValue textbox. Any value typed into this textbox is rendered as HTML.
When dynamically modifying a position attribute, or any other element style, setExpression applies to the style object of the page element:
oBox3.style.setExpression _
"width","cInt(oBox1.value) + _
cInt(oBox2.value)","vbscript"
Here, the width property of the textbox named oBox3 dynamically alters based on the sum of the values in two other textboxes, oBox1 and oBox2. The style object is listed because you're interested in a style property. Set an expression to the value of the width property of the oBox3 textbox. The expression that dynamically sets to the width property is the sum of the contents of oBox1 and oBox2. The language of the expression is VBScript.
You have many other layout possibilities with setExpression. You can even move HTML elements, such as <DIV> tags. This code shows moving a <DIV> tag based on the location of another <DIV> tag:
div1.style.setExpression("posLeft"," _
div2.style.posLeft+div2.clientwidth", _
"VBScript")
You can also create dynamic properties in HTML tags, using the STYLE property. This code uses the STYLE property of the <DIV> tag to accomplish the same results as the preceding code:
<DIV ID=div1 STYLE="left:function
(div2.style.posLeft+ div2.clientWidth)">
Drag and Drop
While users have had the ability to share content between applications with drag-and-drop for the majority of Windows' practical life, Microsoft has now extended the IE object model to provide this functionality from within IE. You can now build applications that enable users to drag content between frames and even to other applications (see Figure 3). IE5 makes incorporating custom drag-and-drop or editing functionality in your Web applications straightforward with an extensive data transfer implementation. An important part of the data transfer object model is the new datatransfer object and its supporting cast of properties and methods. The datatransfer object is available as a property of the window event object, so call it like this:
event.datatransfer.setData("Image")
|
10 Great IE5 Features |
|
This article covers a few of Interrnet Explorer 5.0's many new features. Here are a few more of IE's important new capabilities
Read entire sidebar.
|
The setData method associates source object text with a destination object. Using this method, you can write code that displays text in a field based on the object dropped on it. In fact, with just a few lines of code, a container can transfer its contents to another location. This code shows another example of the setData method in action:
window.event.datatransfer.setData
"Text", OBjProduct
The datatransfer object acts as the conduit for transferring information between the data source and the data target. The data source can provide both data and a data format. In the same way, a target object can request data of a specified format. The datatransfer object's setData and getData methods are central to these exchanges of information.
With this data transfer functionality, you can enable drag-and-drop operations on just about any object. For example, you can write online shopping cart applications that display a catalog and an order form, enabling the users to drag the image of the item they want to purchase and drop it onto the order form.
Two properties unique to drag-and-drop coding in IE5 are effectAllowed and dropEffect. effectAllowed stipulates whether the selection is copied, moved, or linked when dropped. dropEffect matches the system pointer to the action set in effectAllowed.
All the data transfer communication takes place during events. IE5 delivers a rich set of notification events, all of which bubble. Event bubbling refers to the characteristic of events that enables them to be handled both by the object the event applies to, and to the container of that object. For example, if a user clicks on a textbox, an onclick event fires for both the textbox and for the document body, and can be handled in both places. You can modularize your code by trapping these events once they bubble up to the container level.
This code can help you determine if a running instance of IE supports drag-and-drop. If the running instance of IE supports it, window.event.datatransfer returns TRUE. If the running instance of IE doesn't support it, window.event.datatransfer returns FALSE:
If Not IsObject( _
window.event.datatransfer) Then
msgbox ("This version of IE " & _
"does not support Drag and Drop")
Exit Function
End If
Follow the currentStyle
The new currentStyle object exposes the style used to render page elements. This object includes every style property, including Color, Cursor, and fontSize. The currentStyle object returns either the most recently applied element style or the style used to render the element, depending on which was most recently set. Where element properties are not explicitly set, default values are used. These default values render the element and are returned as part of the currentStyle object. When the style has been explicitly set, the style and the currentStyle are the same for a given property (Color, Cursor, fontSize, and so on).
The currentStyle object is similar to the style object, but the style object exposes only style attributes set directly on an element. The sample application includes a designer, named CurrentStyle, which demonstrates the syntax of the currentStyle object. This page explicitly sets some style properties for page elements and relies on default styles for others. It also enables the user to click on various page elements and view and change various style attributes for page elements (download the sample application from DevX; see the Download Free Code box for details).
IE is evolving into a stable, mature platform on which robust business applications can run. More than that, the technologies we see today in IE5 might be the precursors to technologies that might one day enable an HTML-like language to drive the entire Windows user interface. At least one IE5 dialog box (Organize Favorites) is created with DHTML. Today's IE delivers increased performance and stability through fixed-layout tables, an enhanced rendering engine, and improved CSS support. And you can take advantage of IE's new features from within VB. VB6's DHTML Behaviors provide a powerful mechanism for bringing encapsulation and extensibility to Web development. With this level of technology at our disposal today, I can't wait to see what tomorrow has to offer.
Charles Caison is an instructor with DBBasics Corp. (http://www.dbbasics.com) and coauthor of the upcoming Professional ADO and RDS with ASP (Wrox Press). He also speaks at Microsoft Developer Days and other industry events. Reach him through e-mail at charlesc@dbbasics.com.
|