Building Dynamic Visual Basic Programs with DHTML
David Stutz
Microsoft Corporation
February 1998
Many programmers using the Microsoft® Visual Basic® development system and Visual Basic for Applications already use Web infrastructures such as HTML, MIME, and HTTP to reduce the amount of code that they must write to solve common programming problems. Productivity gains associated with Web infrastructure include lower-cost application delivery and maintenance, ways to utilize Internet availability and bandwidth, middle-tier deployment solutions, and, perhaps most importantly, the nearly universal presence of the Web browser as a simple, universal user interface.
With Dynamic HTML (DHTML), Microsoft has pioneered a new and entirely different way to use HTML. No longer is it limited to describing browser "content." Instead, DHTML now forms the basis for a broad new programming model that can provide a rich, interactive UI for any application for the Microsoft Windows® operating system. DHTML can be used to produce customized client/server front ends not only in so-called "thin client" scenarios, but also in traditional EXE-based clients. Programmatic manipulation of page elements is necessary for pages to become truly "dynamic," and since those elements are exposed through OLE Automation, harnessing the power of DHTML is especially easy for programmers.
Rich Declarative Formatting
Programmers can declaratively describe the appearance of a form, a help page, a control, or other programmatic visual element using DHTML's simple, text-based file format. Because this description is declarative, many lines of code that might have existed to handle resizing, positioning, and layout can remain unwritten! This description language is very flexible, and can be used to design resolution and locale-independent visual regions within an application that can be mixed and matched with more traditional Microsoft Win32® regions. Here are some of the more significant formatting features:
- Dynamic layout and resizing behavior. The layout capabilities, dimensional units, and typographic support built into the DHTML page-description format are far richer than those built into standard Visual Basic forms. Layout of pages is defined by the interaction between two kinds of elements: inline elements and block elements. Inline elements can wrap around block elements, and blocks themselves can be positioned using relative, absolute, or percentage dimensions. Both inline and block elements can be nested arbitrarily; in addition, there are many ways to influence element flow, which are beyond the scope of this article. To understand the subtleties of DHTML layout management, consult the Microsoft Internet Software Development Kit (SDK).
- Table-based layout. A special case of the flow-and-block layout mechanism is nested tables. A table is a collection of rectangular regions. Each region can have its own size, color, background texture, and so on. Tables display many of the same characteristics as other block elements, but they also represent a reasonable alternative for displaying more regularly structured data. The dynamic, relative resizing of regions as well as the rich formatting capabilities differentiate table-based layout from the simple two-dimensional positioning available in Visual Basic today. Taken in conjunction with nested flow-and-block positioning, complex layouts can be managed with very little code.
- Scrolling and bottomless forms. In Visual Basic today, scroll bars on a form and complex repeating visual regions within a form must be managed entirely from code. This is not true in Dynamic HTML, where forms are naturally "bottomless" due to the dynamic nature of the medium, and repeating regions are simple to express in a declarative way by using tables or frames.
- Rich typographic support. All Dynamic HTML block elements have margins, borders, and padding, and many can nest other elements and text within themselves. In addition to these formatting options, text contained within Dynamic HTML can have interesting spacing and appearance properties. Developers using Visual Basic can control the baseline-relative positioning, leading, letter-spacing, colors, fonts, indentation, strike-through lines and bullets displayed in their application. Since Dynamic HTML allows text to flow behind, around, or over other elements, and since all of the properties of the text and of the relevant elements can change dynamically, striking visual effects are possible without massive amounts of coding.
- More measurement units. Dynamic HTML supports several different measurement units, including inches, centimeters, points, and device-dependent pixels. In addition, because some properties can be related through their relative position in the page (by containment or property inheritance), percentage values are often useful in combination with these units. Percentage units can enable dynamic layout behavior when a screen region is resized. Developers can use the measurement features in Dynamic HTML to simplify creating a resolution-independent user interface (UI).
- Built-in graphics types. Dynamic HTML supports an interesting and growing list of graphics types, including JPG, GIF, MPG, and PNG, and the handlers for these are built into the package. In Visual Basic, support for new graphics types must usually come in the form of third-party controls, which lead to setup and maintenance complexity.
- Style sheets. Style sheets and the <STYLE> tag can be used to apply a collection of property values to objects represented by other Dynamic HTML tags. Any element of the page can have styles applied to it, either directly or by name. Styles can be applied to multiple elements, and can be changed dynamically at run time. Styles are a complex subject, and entire books have been written about the mechanism, but they are also one of the most powerful and liberating features of DHTML.
- Multimedia effects. Finally, dynamic HTML has the ability to animate elements, to attach one or more filters and multimedia effects to regions of the page, and to sequence these actions in complex ways. In Visual Basic, elements can be animated, but it is not easy to apply transformations to them. Using filters, transition effects, built-in graphic types, and the multimedia controls that come as part of Dynamic HTML, developers can create much more sophisticated animations than with Visual Basic alone.
Object Plumbing Features
There are several unique facets to Dynamic HTML and its object model (defined here as the collection of objects that collectively represent a page) that do not exist as general features of other familiar COM object models such as Visual Basic forms or Microsoft Office documents. These features—event bubbling, generalized event canceling, styles, and property inheritance—were designed as productivity-enhancements for Dynamic HTML and are quite powerful. Like all power tools, when they are misused or misunderstood, they can cause quite a mess. At the very least, these new concepts must be learned and understood by programmers using Dynamic HTML since they are integral features of the object model.
- Event bubbling. Events raised by visual Dynamic HTML elements are propagated through the structural hierarchy of the document, which means that multiple objects can share an event handler, and that multiple event handlers can be called for a single event. Both of these concepts are profoundly different than the current state of the art in Visual Basic, where event handlers are always tied directly to the object raising the event.
- Event canceling. Dynamic HTML events that are propagated along the page's tree of tags can be canceled by the event handlers being used. In addition, some of the events in DHTML occur before the default behavior of the browser is invoked; in these cases, the default behavior itself can be cancelled. Event bubbling and default behaviors are two distinct mechanisms and can (and should) be used independently of each other. Events that precede a default behavior can cancel that default action by setting the ReturnValue property of the global Event object to FALSE, while the CancelBubble property of the global Event object can be set to TRUE in order to stop event bubbling. These features can be used to interpose dynamic behavior based on context, and can be used to implement all sorts of cooperative behaviors.
- Styles. Styles are both an HTML tag and a writing style for HTML—they represent separable "bags of property values" that can be applied to objects in very flexible ways. To the programmer, they are significant because a single style can be applied to multiple objects dynamically, changing all of the objects' appearance or behavior in a single operation. Multiple styles can also be applied to a single object in sequence.
- Property inheritance. Styles and the style-sheet mechanism in Dynamic HTML support multiple ways to inherit property values. Multiple styles can be combined and applied to a single object, using a complex set of combination rules, which allows the programmer to factor UI feedback in powerful (and barely debuggable) ways.
Page Structure
There are several HTML tags and attributes that are important to programmers using DHTML. The ID attribute, which can be applied to any element, is the most important, since it is easiest and safest way to refer to an object in the page. Notice that the ID attribute is used rather than the NAME attribute—this is particularly important to note for programmers using Visual Basic, who are used to the utilizing the Name property of objects for this purpose. Here are several other important ways to create structure within a page:
- DIV and SPAN. The DIV and SPAN tags are "marker" tags that can be inserted into a page's Dynamic HTML. They are often used to apply styles to an arbitrary region, or to mark a region of HTML for manipulation by code. Note that DIV and SPAN regions can have event handlers associated with them; this allows the programmer to create synthetic programmable regions on a given page.
- FRAMESET, FRAME, and IFRAME. Frames represent rectangular regions of a Web page that contain their own encapsulated Web page. Frames are filled in the same way that pages are filled, by using references to URLs. There are two kinds of frames, IFRAMES (the "I" stands for "inline") and FRAMES. The differences between them are in the way that they are hosted, displayed, programmed, and laid out. IFRAMES behave like block elements in the page, while FRAMES behave according to their own tiling rules, and occupy the entire visual region of a page.
One major use of frames in a pre-Dynamic HTML world was to allow dynamic replacement of regions without a reload of the entire page. Of course, manipulating the Dynamic HTML object model can be used to do this more effectively. In the case that a programmer wants to explicitly exploit a separate URL to achieve encapsulation and reuse (at the cost of a slower load), frames are still useful. Frames are also still useful for incorporating "external" pages into containing Web pages and as an organizational device.
- XML. One other powerful structuring mechanism bears mention: the emerging XML standard. XML allows programmers and Web designers to inject nonvisual information about the structure of a page directly into the page itself using custom tags. For the programmer, XML is particularly useful for attaching structural information to a page that can be used by code. The XML specification defines a syntax for defining custom tags that can represent the schema of the information contained in a page. This schema can be used by code contained in the page or by an external program such as an index server to manipulate the page's contents or link to it. See http://www.microsoft.com/xml/ for details on using XML.
Extensibility
There are a number of different kinds of extensibility built into HTML, which enable programmers and Web designers to inject new behaviors and appearance into their pages without upgrading the browser.
- Objects. The most useful and familiar extensibility mechanism for Visual Basic programmers will be the OBJECT tag, which is used to insert COM objects directly into a Dynamic HTML page. Internet Explorer 4.0 actually knows how to host multiple kinds of COM objects, both visible and invisible, including ActiveX® controls, active documents, and Automation objects. Once inserted with the OBJECT tag, an object can be accessed directly by name, which means that Visual Basic code manipulating a Dynamic HTML page can easily access and manipulate objects within that page directly. Java applets can also be accessed and manipulated in this way.
- Dynamic creation of form elements and content. The set of elements populating a Dynamic HTML page can be changed on the fly, making it simple to create a data-driven or context-sensitive user interface. It is no longer necessary to precreate all of the control elements of a page during design time. Instead, elements can be created and "squirted" into the page's underlying Dynamic HTML. These dynamically created elements can have Visual Basic code attached to their events either through the use of WithEvents variables or through event bubbling. The Dynamic HTML run time takes care of siting the element properly in the container's name space and on-screen visual region. Because Dynamic HTML supports the creation of new elements on the fly, regions can be created and populated by duplicating a "template section" and then filling it with content.
Advanced uses of this capability include the ability to insert elements that are themselves template regions into a page on the fly. Using this method, sophisticated data-driven templates can be deployed and shared across a wide spectrum of uses, reducing the amount of code and data transported across the network. Programs that wish to create forms or content on the fly can do so by injecting objects into the page at run time; a form might be created based on a description of its fields in a database, for example.
- Mime handlers. The most venerable form of extensibility within HTML is known as "MIME handlers." Using this mechanism, subelements of an HTML page can have helper applications associated with them; these helpers take care of reading, displaying, and executing the content associated with the subelements involved. This is a coarse-grained extensibility mechanism that will continue to be useful; especially as new MIME types emerge over time and are supported by Microsoft Internet Explorer. Every MIME handler that is plugged into the browser is a reusable chunk of code for handling the media type represented. Of course, this translates to less code to write for application programmers!
Scripting
In addition to the rich declarative formatting and programmer-defined structure that Dynamic HTML can express, DTHML also allows developers to customize their pages through scripting. Developers can insert script directly into the body of a document, and this script can, in turn, manipulate all of the objects that represent the page (as well as its container). Immediate load-time execution of this script is possible, but this is a particularly error-prone and difficult-to-understand technique to use effectively. Because of this, the familiar concept of event handlers is the preferred mechanism for attaching behavior, whether in compiled code or in script, to a page.
Complex tasks can be accomplished using script alone. This is not always the best approach, however, since the "in the page," late-bound nature of script makes it hard to read, hard to structure for reuse, and hard to protect as intellectual property. It also adversely impacts the debuggability and maintainability of a page, and can be slower than compiled code in several common scenarios. By all means, don't forget that using compiled code in conjunction with DHTML is often a reasonable approach, packaged either in the form of OBJECT tags on the page or in the form of the Microsoft WebBrowser control.
There are positive aspects to script, including good network round-trip characteristics, malleability, and the quick load times that characterize simple pages. Script is also a cross-browser, cross-platform, programming solution. Understanding when to use script versus code is one of the most difficult aspects to Dynamic HTML, since there is so much overlap. It is possible for a careful programmer to write good script, and the technical problems mentioned above eventually will be addressed through better script programming tools. It is certainly safe to say that Microsoft Windows–applications programmers using DHTML to extend their applications using the techniques described in this article are better off using compiled code for the bulk of their development.
Program Execution
The final characteristic to be noted in this programmer-centric whirlwind tour of Dynamic HTML is the asynchronous nature of the medium. Even plain old nondynamic HTML has the notion of hyperlinking and asynchronous downloading built-in to the format. There is no code necessary to navigate between pages! Of course every feature comes with a dark side. Programs that use HTML to represent their UI must be prepared for the page to be only partially loaded when it is used, and for control to shift to other pages that are exposed as a hyperlink either through HTML tags or through code.
This "load-as-you-go" style of programming is not new to programmers using Visual Basic, who have been handling events asynchronously since Visual Basic version 1.0. It certainly has implications for building up and tearing down resources associated with the page, however, since this model suggests that resources should be provided in a "just-in-time" lazy fashion. Even in the case where a page is not usable until it loads in its entirety, asynchronous behavior can still be helpful since the user interface remains responsive during load.
HTML has other interesting execution characteristics that are best left alone. As mentioned above, script can be sprinkled into an HTML page at arbitrary locations, for example, and when such "immediate" script is located, it is executed, as the name implies. Since this happens at page load time, very strange and complicated asynchronous behavior can be created, including self-modifying content. The ability to replace elements is similar—some tags, such as images, are loaded asynchronously by the browser. Because of this, the structure and appearance of the page changes as these "replacing elements" appear and are loaded. This is a very important optimization for slow links, but it can cause user-interface distraction when the page is being used in a more traditional way. DTHML programming offers limitless opportunities to Keep It Simple!
Accessing the Dynamic HTML Object Model from Within Visual Basic 5.0
In order to try the many Dynamic HTML programming ideas outlined above, you first have to understand how to access the Dynamic HTML object model from within the WebBrowser control in Microsoft Visual Basic 5.0. In essence, you need to join the Web Browser object model to the Dynamic HTML object model that is a part of Internet Explorer 4.0.
Visual Basic 5.0 doesn't make it obvious how to join them, but it's easy to do. The following steps will help you create a template that you can use to build your own user interface (UI) using Dynamic HTML.
Note You need to be running Visual Basic 5.0 with Service Pack 2 or later installed, and you must have Microsoft Internet Explorer 4.0 installed to complete the following procedures.
- Create a Visual Basic project (my sample is named WebEXE.vbp), and open a blank form.
- Click Components on the Project menu, and then select Microsoft Internet Controls to add an instance of the WebBrowser control to your form. The Microsoft Internet Controls box should now appear, and be checked, in both your Components and References dialog boxes.
- Click Preferences on the Project menu. Click Browse and locate the Mshtml.dll file in your Windows system directory, select it, and click Open. This file will appear in the References dialog box as the Microsoft HTML Object Library.
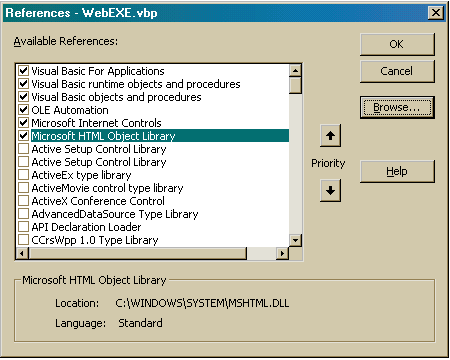
Figure 1. Adding a reference to a Visual Basic project
The Microsoft Internet Controls and Microsoft HTML Object Library references should both be checked in your References dialog box.
- Define a variable that will hold a reference to the Dynamic HTML document:
Dim WithEvents myDoc as DHTMLDocument
- In the form's load event, write a line of code that uses the WebBrowser control to navigate to a blank page, like this:
WebBrowser1.Navigate "about:blank"
- When this blank HTML page loads, it will fire the DocumentComplete event. At this point, you can take the document property of the WebBrowser control and assign it to your document variable.
Set myDoc = WebBrowser1.Document
Voilà! You now have access to the Dynamic HTML object model.
Using the Dynamic HTML object model, programmers can try event bubbling, programming with typographic content, managed layout, and the other Dynamic HTML ideas described in this article.
The code for the template we created, using the above steps, to access the Dynamic HTML object model is in Figure 2:
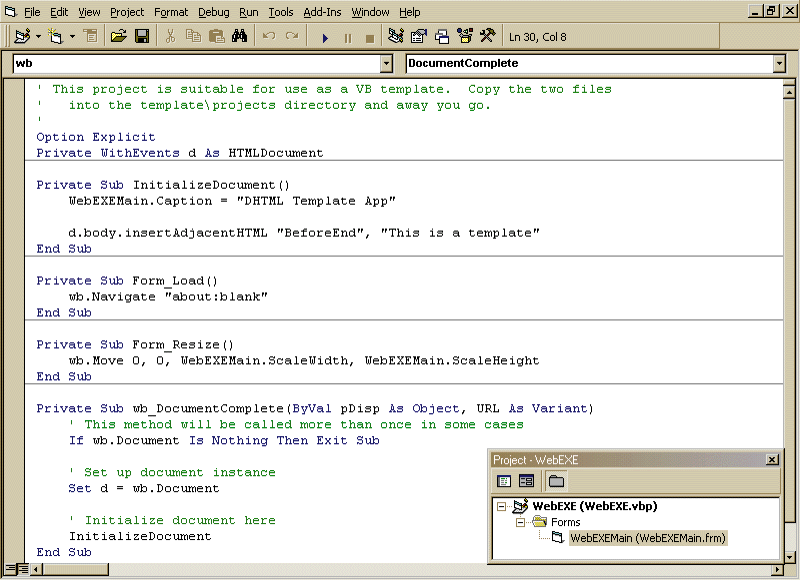
Figure 2. The Visual Basic code used to access the DHTML object model
Final note Programmers using Visual Basic should be aware that there is another important way to access the Dynamic HTML object model. Rather than using the WebBrowser control, you can also build a UserControl that accesses the DHTMLDocument through its Parent property. In this case, from your UserControl, the joining of the object models is accomplished in this way:
Set myDoc = Me.Parent
Once you get hold of the Dynamic HTML Document, the sky is the limit! There are numerous rendering and interaction techniques waiting to be exploited.
Conclusion
Web technology is rapidly becoming an indispensable tool for developers. On client computers, the technology affords a very rich user interface for distributed computing with minimal setup impact, while on the middle-tier or server it represents a flexible, yet simple, way to factor applications into data, style, navigational logic, and UI, and then to independently manipulate these entities.
DHTML augments and complements the capabilities of Visual Basic and Visual Basic for Applications, and because the programming model is exposed through Automation, it can easily be used in combination with existing features. Visual Basic programmers who learn to use Dynamic HTML will utilize their existing skills while building applications that integrate new Web infrastructure. The combination of these two technologies is a powerful and productive way to create programs.